A data structure is taking data and organizing, managing, or storing it in a format so it can be easily accessed and modified. Performing specific operations includes accessing, modifying, or even deleting that data.
So below is a very simple definition of data structures and algorithms and how they are related to each other:
- A data structure is about organizing and managing data effectively so that we can perform specific operations efficiently. An algorithm is a step-by-step procedure to be followed to reach the desired output.
- Algorithm steps use one or many data structures to solve a problem.
Great, now that we have a basic understanding of the two different, but related items, below is a list of common data structures you will encounter in order to tackle those oh-so-fun algorithms!
First let’s understand that there are different kinds of data structures that are suited for different kinds of applications and they are broadly classified as linear and non-linear data.
Linear Data Structure has data elements arranged sequentially and each member element is connected to its previous and next element. Traversing a data structure or iterating over the data structure, which means “visiting” or “touching” each element of the structure, is sequential. These data structures are easy to implement because computer memory is also sequential. Examples of linear data structures are arrays, linked list, stack and queue.
Non-linear Data structures are not arranged sequentially or linearly. Each element can have multiple paths to connect to other elements. You cannot iterate or traverse all the elements in a single run. Non-linear data structures are not easy to implement but are more efficient in utilizing computer memory. Examples of non-linear data structures include trees and graph.
Let’s briefly explore the various data structures:
Array
- An array is the simplest type of data structure that holds elements of the same data type (integer, float, string, etc.)
- It is a linear data type
- Data stored in each position of an array is given a positive value called the index.
- The index value starts with 0 for the first element in an array.
Say we have the prices for 6 grocery elements and their index position. We can store all the integers in an array.

This array can be written as such:
const arr = [10, 5, 23, 8, 12, 7]
Linked List
- A linked list is a type of data structure consisting of nodes and each node “knows” the address of the next node. A good example of this would be connecting the dots in order to create a picture. You know how to connect the dots because they are numbered.
- A linked list is a sequential collection of elements. The element is stored in the form of nodes which consist of two key pieces of information — the data value itself and a pointer / next that references the next node in the list.
- Data stored in a linked list might be of any form, strings, numbers, or characters.
- Linked lists are not great for getting direct access to an element like an array but they are really efficient for inserting and deleting elements because of the way their elements are stored in memory.
- There are two types of linked lists — a singly linked list where each node has one pointer to the next node in the list. There is also doubly linked list where each node has a pointer to the next node and a second pointer of the previous node.

The main properties of a linked list data structure are:
– size: The number of elements in the linked list
– head: The first element in the linked list
– tail: The last element in the linked list
- The first node (containing 2) is called the Head Node because there isn’t any node pointing to it.
- Node 2 stores the address of the node containing 4, and so on.
- The Tail Node points to null, which indicates the end of the list.
Some practical applications of the linked list include:
- Images are linked with each other so that an image software uses a linked list to view the previous and next images.
- Music players also use a linked list to switch between music and often a circular linked list is used.
If you want to delve more into linked lists, check out these great resources:
NoobCoder — Linked List in JavaScript for Beginners
Beiatrix — Linked Lists | Data Structures in JavaScript
Hash Table
- Data structure that allows you to create a list of key-value pairs. You can then retrieve a certain value by using the key for that value.
- Hash table is a data structure that uses hashing to implement associative arrays or mappings of key value pairs
- A hash function or hashing takes in a string, converts it into some sort of integer or a string of letters and numbers, and then remaps that integer into an index.
- A hash table begins with multiple placeholders called buckets that will hold content. To add any key value pair to the hash table, you take a key and pass it through the hash function that outputs a number that corresponds to an index in an array where we will store the value
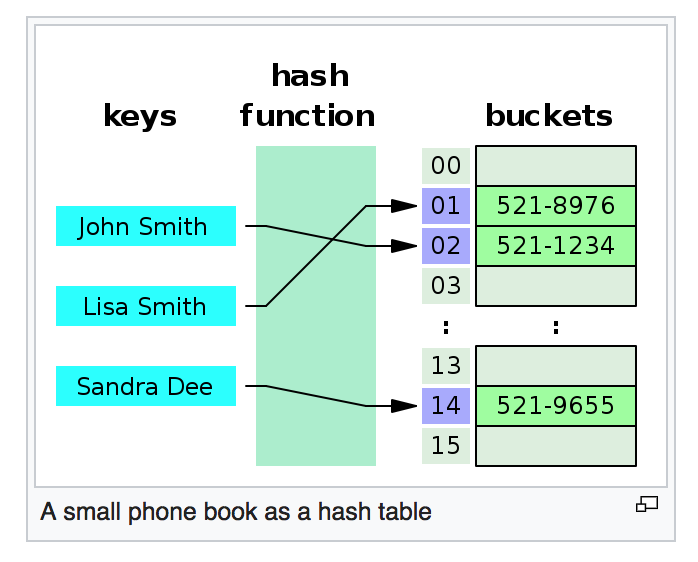
If you want more information about hash tables. The resource below dives deeper into the topic:
ComputerScience — Hash Tables and Hash Functions
Stack
- A stack is a data structure that helps organize data in a particular order and follows the “last in first out”(LIFO) principle. This means the last element inserted inside the stack is the first to be remove.
- Think of stack as a pile of books where you can only take the top item off the stack to remove books from it and you can only add a new book at the top. This type of data accessing is called “last in first out”.
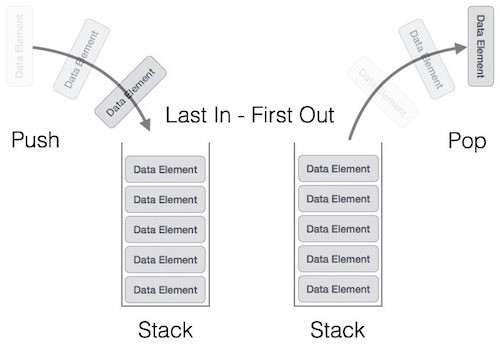
Queue
- A linear data structure that follows the “first in first out” (FIFO) principle. The element inserted first is the first to be removed.
- Common examples of a queue is lines of people waiting for a service or to retrieve something. The first person in the line is served first while the person who is last on the line is served last.

Graph
- A non-linear data structure that consists of a finite set of vertices (or nodes) and a set of Edges that connect a pair of nodes
- Graphs are used to represent networks such as paths in a city or telephone network. They are also used in social networks Facebook and LinkedIn.
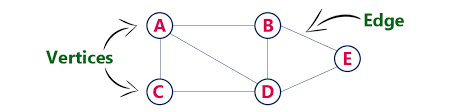
- In the above diagram:
Vertices = {A, B, C, D, E}
Edges = {AB, BE, ED, DC, CA, AD, BD}
Tree
- A non-linear hierarchical data structure that consists of nodes connected by edges. Each node contains a value or data, and it may or may not have a node child.
- Examples of hierarchical organization is a family tree, in HTML — the Document Object Model (DOM) works as a tree.
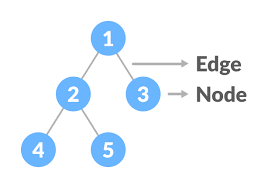
There you have a basic overview of the different types of data structures that you can use in your algorithms to solve various problems!