JavaScript has been a major player in web development for many years now, and it’s only getting more and more popular. With the increasing complexity of web applications, it’s becoming increasingly important to understand how to write asynchronous JavaScript, especially when working with APIs.
Asynchronous programming is a technique for executing multiple tasks simultaneously, instead of waiting for one task to finish before moving on to the next. It allows for multiple operations to occur at the same time, which can greatly improve the performance of a web application. APIs are a way for different software applications to communicate with each other, and they play a big role in the functionality of many modern web applications.
In this article, we’ll explore asynchronous JavaScript in-depth and learn how to work with APIs. We’ll cover the following topics:
- Understanding asynchronous programming
- Callbacks
- Promises
- Async/Await
- Working with APIs
Understanding Asynchronous Programming
In a synchronous programming model, the code is executed one line at a time, in the order that it’s written. This means that the program will block, or wait until a task is complete before moving on to the next task. This can lead to slow performance and a poor user experience, especially in web applications.
With asynchronous programming, tasks are executed simultaneously, which means that the program can continue to execute other tasks while one task is blocked. This can greatly improve the performance of a web application.
Callbacks
A callback is a function passed as an argument to another function, to be executed when the first function is finished. In other words, a callback is a function that is called when a task is complete. This allows for the execution of multiple tasks simultaneously, as one task can be executed while the other is waiting for its callback to be executed.
Here’s an example of a simple callback function:
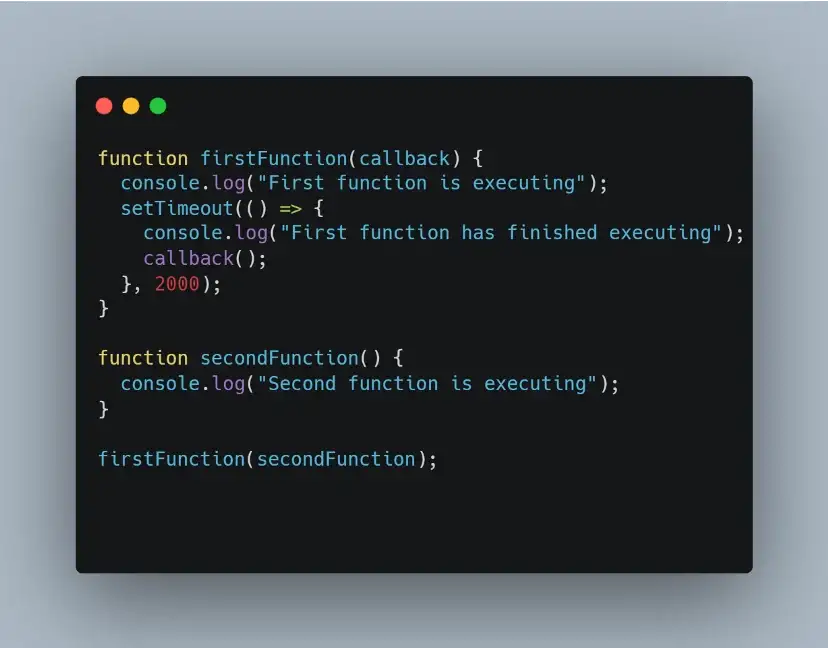
In this example, firstFunction
is executed first, and secondFunction
is passed as a callback. firstFunction
uses setTimeout
to simulate a delay, and when the delay is over, it logs a message to the console and then calls secondFunction
. secondFunction
logs a message to the console and is then executed.
This example demonstrates how callbacks can be used to execute multiple tasks simultaneously.
Promises
Promises are a way to handle asynchronous operations in JavaScript. A promise is an object that represents the eventual completion or failure of an asynchronous operation. Promises have three states: pending, fulfilled, and rejected.
Here’s an example of a simple promise:
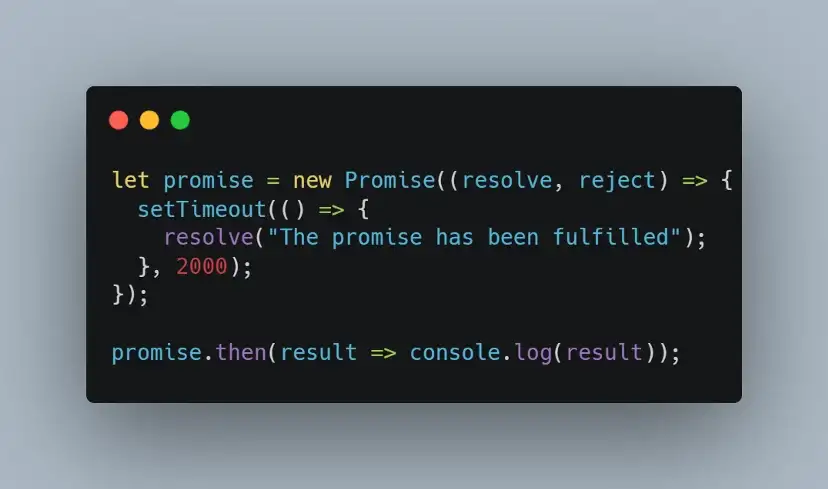
In this example, a new promise is created using the Promise
constructor. The resolve
function is called when the asynchronous operation is complete, and the reject
function is called when the operation fails. In this case, we’re using setTimeout
to simulate a delay, and when the delay is over, the promise is resolved with the message “The promise has been fulfilled.”
The then
method is used to handle the resolution of the promise. In this example, the then
method takes a callback function as an argument, which is executed when the promise is resolved. The resolved value is passed to the callback function as an argument, and in this case, it’s logged to the console.
Promises are a powerful tool for managing asynchronous operations in JavaScript. They provide a way to handle the resolution or rejection of asynchronous operations, which can make your code more readable and maintainable.
Async/Await
Async/await is a modern way to write asynchronous code in JavaScript. It’s a combination of asynchronous functions and promises, and it provides a way to write asynchronous code that looks like synchronous code.
Here’s an example of asynchronous code written with async/await:
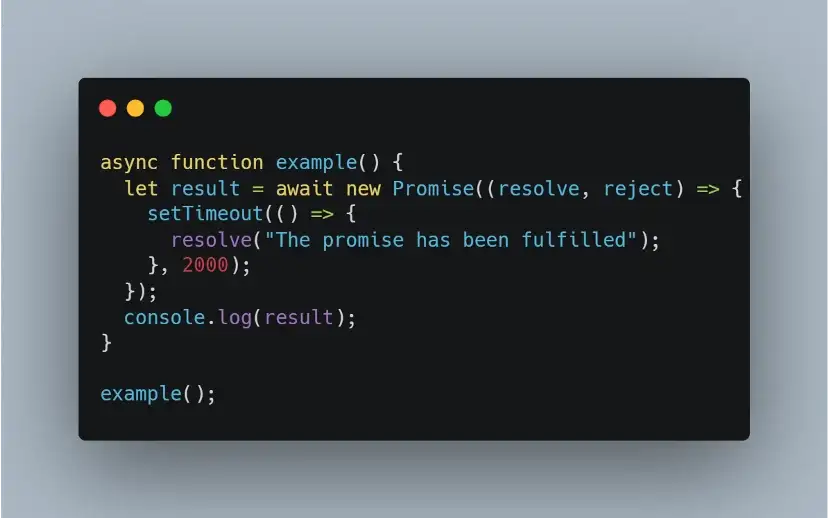
In this example, the example
function is declared as asynchronous using the async
keyword. The await
keyword is used to wait for the resolution of the promise before moving on to the next line of code. In this case, the resolved value of the promise is stored in the result
variable, and then logged to the console.
Async/await provides a clean and concise way to write asynchronous code, and it can make your code much easier to read and understand.
Working with APIs
APIs, or Application Programming Interfaces, are a way for different software applications to communicate with each other. They provide a standardized way for one application to access the functionality of another application, and they play a big role in the functionality of many modern web applications.
Here’s an example of how to make a simple API request using JavaScript:
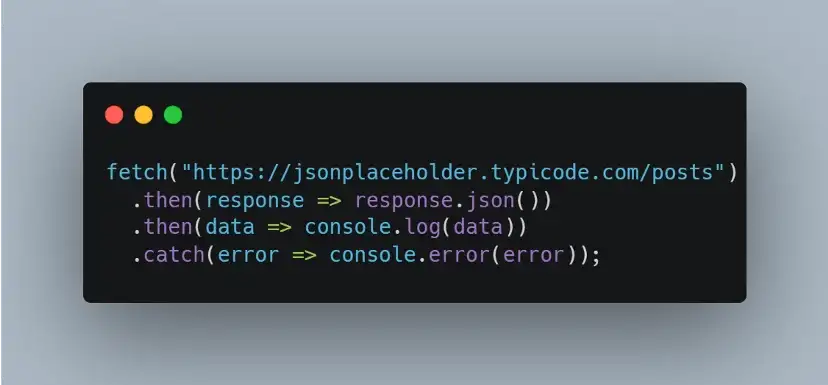
In this example, we’re using the fetch
method to make a request to an API endpoint. The fetch
method returns a promise, and we’re using the then
method to handle the resolution of the promise. The first then
method takes the response from the API and converts it to JSON using the json
method. The second then
method takes the data from the API and logs it to the console. The catch
method is used to handle any errors that might occur during the API request.
Working with APIs can be challenging, but understanding asynchronous programming and the tools that are available, such as callbacks, promises, async/await, and the fetch
method, can make it much easier.
In conclusion, asynchronous programming and APIs are an important part of web development, and it’s essential to understand how to work with them in order to create fast and efficient web applications. With the knowledge gained from this article, you’ll be well on your way to creating high-quality, scalable, and maintainable web applications.