Object-oriented programming is simply a programming pattern that is based on the concept of different types of objects and their properties.
Now, mostly all programming languages support OOP, but in this article, we would look into key characteristics of OOP in javascript and I would ensure to exemplify using simple examples.
We would take a look at two key OOP principles: inheritance and polymorphism on-base level in the sense of how it actually relates to javascript code, using the core characteristics of OOP in javascript to exemplify these principles, we have;
Constructors and the “this” keyword.
The two most essential things in Object-oriented programming in javascript and just about any other programming language is the this
keyword and the constructors
.
While Es6+ is considered a syntactic sugar and provides a more convenient way of writing constructors or declaring classes, I would be making use of Es5 in this article so we can have a deeper understanding of how constructors work under the hood.
Just like object literals where key-value pairs objects are assigned to a variable so does a constructor work but for multiple instances, unlike object literals, constructors consist of prototypes and inheritance.
First, having a constructor of either a single or multiple properties, we can instantiate an object from it using the new
keyword. See code demonstration and console results below:
function Entity(name) {
this.name = name;
} // declaration of a single property "Entity" constructor taking in a "name" argument
const human = new Entity('Creature'); // instantiating using the "new" keyword and assigning "Creature" as a name
console.log(human); // calling instantiated variable
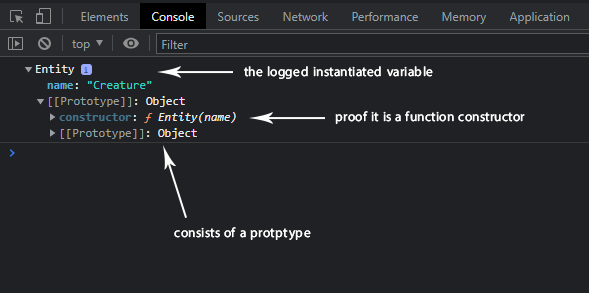
The this
keyword is a very important keyword, its value is determined by the current context in which your code is running. In our example, its value is scoped to the Entity
function context. Whereas if used outside the function scope or in the global scope you would get the window object instead since it now exists in a global context. You can learn more about this
keyword here.
You can add multiple properties to the constructor as well and instantiate them differently.
Prototypes & Prototypal Inheritance
Every object in javascript has a prototype. A prototype itself is an object, and all objects can inherit properties and methods from their prototypes. This means we can as well assign or append our choice of functions into the constructor prototypes chain.
See code representation below:
function Entity(name, dod) {
this.name = name;
this.creationDate = new Date(dod);
} // declaration of a multiple property "Entity" constructor
Entity.prototype.getTotalYears = function() {
return new Date().getFullYear() - this.creationDate.getFullYear();
} // inputing the "getTotalYears" function into the "Entity" constructor's prototype
const human = new Entity('Creature', 'january 09 2000'); // instantiating using the "new" keyword and assigning a name and date
console.log(human.getTotalYears()); // logging the total years of the instantiated variable using the "getTotalYears" function in the prototype
console.log(human); // logging the instantiated variable
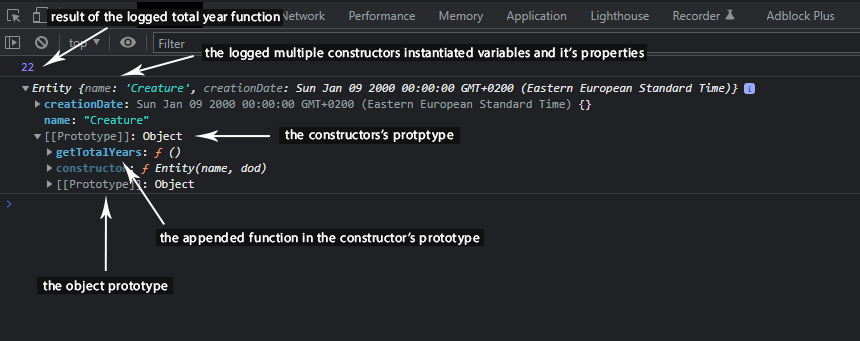
Prototypal Inheritance is when an object inherits its properties from another object in the same code context, this can be achieved with the help of object.create()
method.
See code representation below:
function person(name) {
this.name = name;
} // initializing "person" constructor
person.prototype.greets = function() {
return `${this.name} says hi`;
} // creating a "greets" function for the person constructor and assigning to it's prototype
const Yasir = new person('Yasir'); // instantiating a new student object
function Entity(name, regards) {
person.call(this, name); // calling the "person" constructor and assigning it to the "Entity" constructor
this.regards = regards;
} // initializing "Entity" constructor
Entity.prototype = Object.create(person.prototype); // INHEERITING THE "PERSON" CONSTRUCTOR PROTOTYPE TO THE "ENTITY" CONSTRUCTOR PROTOTYPE
const MrSmith = new Entity('Mr.Smith', 'how are you?'); // instantiating a new "Entity" object
console.log(Yasir); // logging the student object
console.log(MrSmith); // logging the teacher object
console.log(MrSmith.greets()); // calling the "greets()" function from "person" constructor on the "Entity" constructor and printing the result to the console
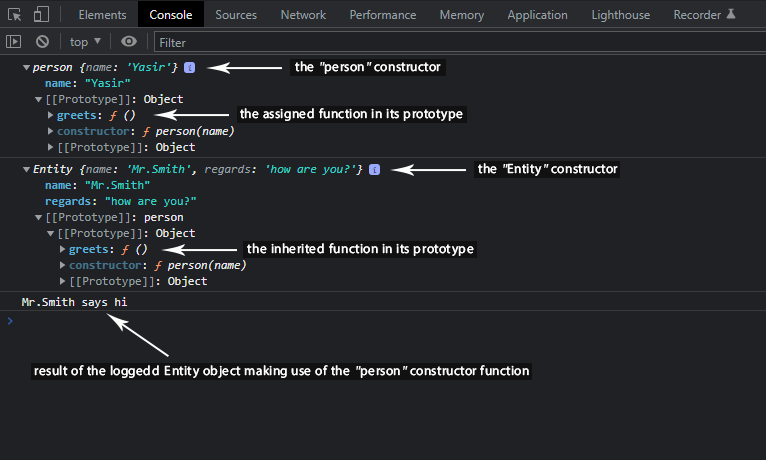
Conclusion
There are core objects that make use of constructors but are not advisable to use, for instance, the Date()
object.
When working with object-literals prototypes are gotten from object.prototype
but when working with a constructor object it is gotten from (the constructor name).prototype
Prototypes can not be accessed by a for-in loop.
The art of appending/assigning functions into the constructor’s prototype is considered efficient, and in good practice, it is advised.
The Call()
method is a function used to call another function from another code block in the same context.